Homework 4: Graphics, Applets and File
I/O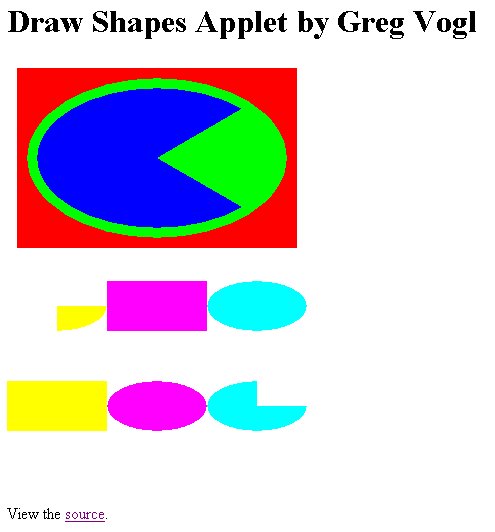
- Review the example graphics files mentioned in
Lecture 8.
- Create a directory named javahw4/ in your directory.
- Copy data files shapes1.txt and
shapes2.txt into your javahw4/ directory.
- Create an HTML document named shapes.html and save into your
javahw4/ directory.
- The title of the document should be "Draw Shapes Applet by Your Name."
- There should be two applet tags.
- Each applet tag should use DrawShapesApplet.class as the code.
- Each applet should have dimensions width 300 and height 200.
- Each applet tag should contain one parameter "filename", the name of a
text file.
- Its value should be either "shapes1.txt" or "shapes2.txt".
- Create a class file named DrawShapesApplet.java and save into your
javahw4/ directory.
- Create the class definition:
class DrawShapesApplet extends Applet
- All other code should go into the
paint()
method of the
class.
- Use the applet's
getParameter()
function to get the name of
the file from the param tag.
String filename = getParameter("filename");
- The applet should open the specified file as follows:
URL fileURL = null;
URLConnection connection = null;
InputStream in = null;
try {
fileURL = new URL(getDocumentBase(), filename); }
catch (MalformedURLException e) { System.out.print(e); }
try { connection = fileURL.openConnection(); in = connection.getInputStream();
}
catch (IOException e) { System.out.print(e); }
BufferedReader reader = new BufferedReader(new InputStreamReader(in));
- Use the
readLine()
function to read each line in the file.
- Use a while loop to loop while the line is not null.
- Wrap the entire loop in a try block and add an empty catch block (as
above).
- Create a
StringTokenizer
object to read each part of the
line.
StringTokenizer st = new StringTokenizer(line);
String command = st.nextToken();
- If the command is "color", use the next three numbers to change foreground
color.
- You will have to get three more tokens and cast to int.
- Else if the command is "line", "rectangle", "oval" or "arc", display the
filled shape with the coordinates listed.
- You will have to cast each of the tokens to ints to get the coordinates.
- Otherwise ignore the entire line.
- Valid commands are as follows (all dimensions in integers):
color
r g b
line x1 y1 x2 y2
rectangle
x y width height
oval
x y width height
arc
x y width length startAngle arcAngle
- The program should work for the two test data files and any other correct
input file.
- No other error or exception handling is required.
- Import the necessary classes:
- Applet from java.applet
- Graphics and Color from java.awt
- InputStream, InputStreamReader, BufferedInputStream, BufferedReader and
IOException from java.io
- StringTokenizer from java.util
- URL, URLConnection and MalformedURLException from java.net
- Compile the applet by typing
javac DrawShapesApplet.java
.
- Open shapes.html to test and debug the applet.
- Use Ark to create an archive of your directory named
javahw4.zip
.
- Send the source code to the
instructor as an attachment in a message with subject java hw 4.