Lab 8: Graphical User Interfaces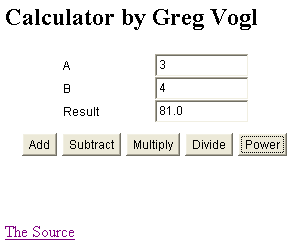
The goal of this exercise is to create a simple calculator. The user
types two numbers into text fields and can click Add, Subtract,
Multiply, Divide, or Power. The resulting calculation is displayed in a third
text field.
- Review Lecture 9.
- Create an empty directory named
javalab8/
in
your directory.
- Browse to the examples in Teach Yourself Java Ch. 12, in the
java1.1 folder.
- Copy
ButtonActionsTest.html
and ButtonActionsTest.java
into
your javalab8
directory.
- Rename your copies to
Calculate.html
and Calculate.java
.
- Change
ButtonActionsTest
to Calculate
in both files using
search and replace.
- Modify the interface in
Calculate.java
.
- Add a label
A
and an editable text field a
of width
10.
- Add a label
B
and an editable text field b
of width
10.
- Add a label
Result
and non-editable text field result
of
width 10.
- Put these components into a new panel
p1
using a grid layout with
3 rows and 2 columns.
- Change the text of the buttons to
Add
, Subtract
,
Multiply
,
Divide
, Power
.
- Make sure the buttons and text fields are instance variables which are
defined in the
init()
method.
- Put the buttons into a new panel
p2
using a centered flow layout.
- Add the two new panels to the applet.
- Compile the applet by typing
javac
Calculate.java
.
- Open
Calculate
.html
to test and debug the applet GUI and
layout.
- Your applet should look like the picture at right.
- Create the event handler in
Calculate.java
.
- Copy the code from
HandleButton.java
into Calculate.java
as an
inner class.
- Make sure to
import java.awt.event.*
at the top.
- Add to the
HandleButton
constructor three TextField
arguments:
a
, b
, result
.
- The
HandleButton
class will also need three
TextField
variables with the same name.
- In the constructor, set these equal to the arguments using
this.a = a
, etc.
- Instead of using a Color variable c, use a
String
variable named
operation
.
- Change the five
HandleButton
method calls to take
this
plus these four
arguments.
- Each button needs its own
HandleButton
object.
- In the
actionPerformed()
method of HandleButton
,
- Remove the statement to set the background color.
- Declare three floating point variables
fa
, fb
, and
fresult
.
- Get the text from the two text boxes using
getText()
methods
and convert to float using Float.parseFloat()
.
- Test the operation variable and add, subtract, multiply,
divide or take the power accordingly.
- Set the result text field to
fresult
using its setText()
method.
- Compile the applet by typing
javac
Calculate.java
.
- Open
Calculate.html
to test and debug the applet event handler.
- Enter two numbers and check that the buttons correctly calculate the
sum, difference, etc.
- Compress the folder using the Ark archiving program to
produce
javalab8.zip
.
- Send the
javalab8.zip
file to gvogl@umu.ac.ug as an attachment in a
message with subject java lab 8.