Homework 5: GUI Components and
Data Structures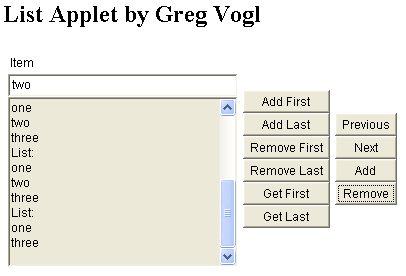
In this exercise you will create an applet with a GUI to manage a doubly linked list
of strings.
- Review Lecture 9, Lecture 10 and Lab
8. Also see the Web Resources JavaScript example list2.htm.
- Create a directory named javahw5/ in your directory.
- Create an HTML document named List.html and save into your
javahw5/ directory.
- The title of the document should be "List Applet by Your
Name."
- Create an applet tag with code ListApplet.class, width 400
and height 300.
- Create a Java applet named ListApplet.java and save into
your javahw5/ directory.
- Create the class definition:
class ListApplet extends
java.applet.Applet
- Create the interface by putting GUI elements in an
init()
method.
- Create a label
Item
.
- Create a text field of width 30 named
item
.
- Create a scrollable non-wrapping text area of width 30 and
height 10 named
items
.
- To reduce the amount of repetitive code, create a method named
makeButton()
.
- Its arguments: a ListApplet, a
Panel, a Button and a String.
- It should create a new button, add an action
listener, and add the button to the panel.
- Create buttons Add First, Add Last, Remove
First, Remove Last, Get First, Get Last.
makeButton(this, panel2, addFirst, "Add First");
- Create buttons Previous, Next, Add and Remove.
- Add these 13 GUI elements to a layout manager.
- Group buttons by adding them to panels, then adding the
panels to the applet.
- The label, text field and text area could go in a panel with
BorderLayout.
- The first six buttons could go in a panel with GridLayout of 6 rows
and 1 column.
- The remaining four buttons could go in a panel with GridLayout of 4
rows and 1 column.
- Each GUI element needs to be a class variable (declared outside any
method).
- Import the necessary classes from
java.awt
and
java.awt.event
.
- Save changes and compile by typing
javac
ListApplet.java
- Test by opening List.html in a browser.
- Create a linked list and list iterator in
init()
.
- The first six buttons are handled by a
LinkedList
object.
LinkedList myList = new
LinkedList();
- The other four buttons are handled by a
ListIterator
object.
ListIterator myIterator
= myList.listIterator();
myList
and myIterator
should be class
variables (declared outside any method).
- Import the necessary classes from
java.util
.
- Create a method named
showList()
to
display the items in the text area.
- Add "List:" to the text area using
items.append("List:\n");
- Create a
ListIterator
named i
from the linked
list.
- Loop through the list items while
i.hasNext()
is true.
- Add each to the text area using
items.append(i.next().toString()
+ "\n");
- Handle the events.
- Add an inner class named
HandleButton
which implements
ActionListener
. HandleButton
needs six class variables and constructor
arguments:
ListApplet, TextField, TextArea, LinkedList, ListIterator, String
- In the constructor, set each class variable equal to its corresponding argument, e.g.
this.app = app;
- All the following actions are handled using
if
statements
in an actionPerformed()
method. - If the user clicked an Add button, an element should be added
to the list.
- Add First adds an item to the beginning of the list; Add Last
adds to the end.
myList.addFirst(item.getText());
- Add uses the
ListIterator
and adds the text field
item at the current
position.
- If the user clicked a Remove button, an element should be
removed if one exists, i.e.
myList.size() > 0
- Remove First removes the item at the beginning of the list;
Remove Last removes the item at the end.
- Remove uses the
ListIterator
and removes the item at the
current position.
- Note:
remove()
only works after a call to previous()
or
next()
.
- If the user clicked Previous or
Next, the iterator should move to the previous or next item
- First test if a previous or next item exists, i.e.
myIterator.hasPrevious()
- If the user clicked a Previous, Next, Remove or Get button,
the element should also be displayed in the text field.
item.setText(myIterator.previous().toString());
- Whenever any button is clicked, call
showList()
.
- Save, compile and test. Test the first six buttons separately from the
other four.
- Use Ark to create an archive of your directory named
javahw5.zip
.
- Send
javahw5.zip
to
the instructor as an attachment in
a message with subject java hw 5.